Problem Statement
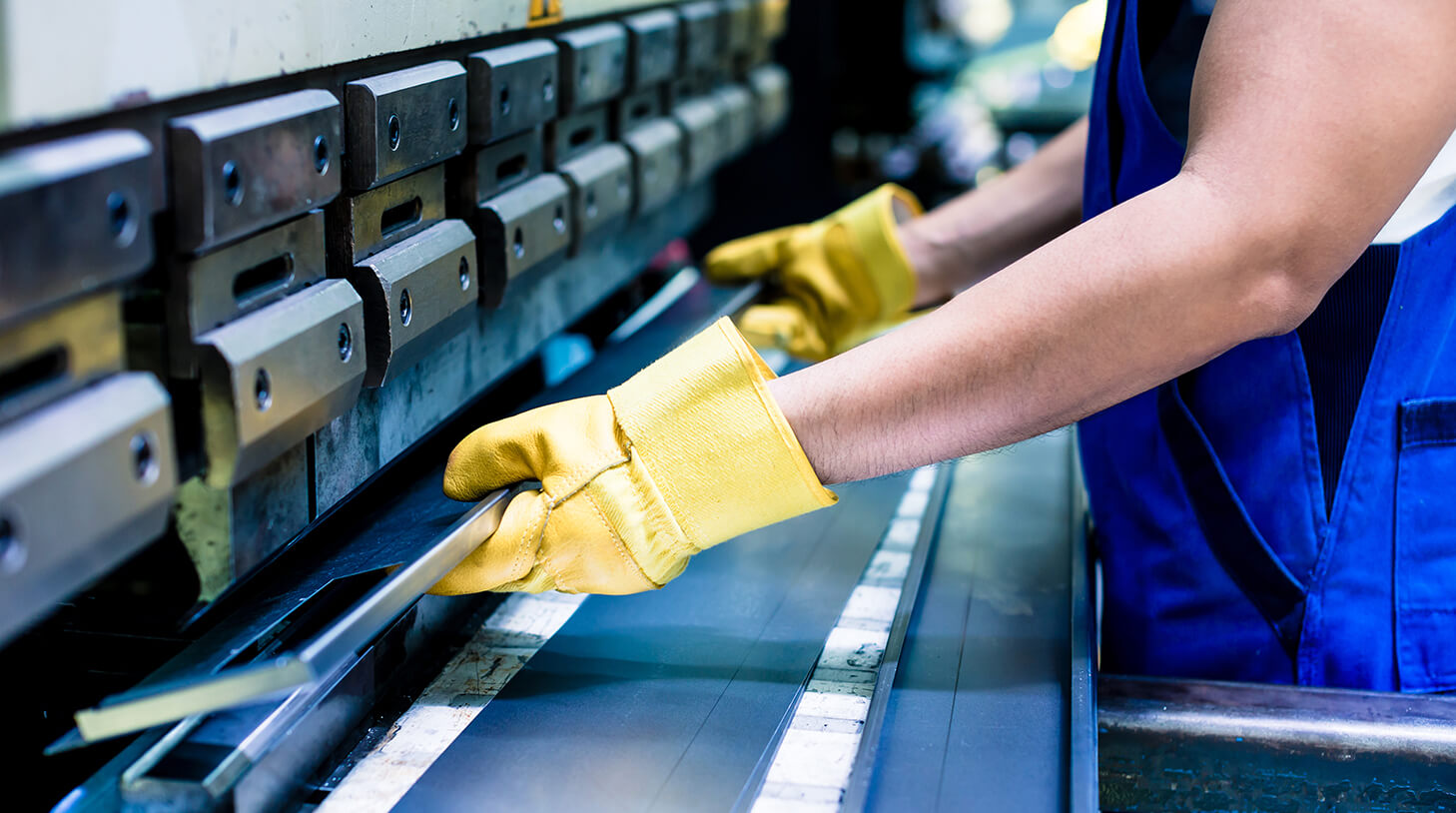
A factory production line has multiple tasks
that need to be performed in a specific order.
Each task has an estimated duration and priority
level. To optimize production, the factory wants
to ensure that tasks are scheduled efficiently based
on priority and duration.
You are tasked with designing a linked list structure
to manage the tasks in the production line. Each task
in the list should be represented as a node in the linked list,
which contains the task name, duration, and priority. Your goal
is to build functions to add, remove, and reorder tasks based on
certain criteria.
Requirements
Create a Linked List Node Class. Each node should contain:
- task_name (string): the name of the task.
- duration (int): the duration of the task in minutes.
- priority (int): the priority of the task (1 is highest priority, higher numbers are lower priority).
- next (pointer): a reference to the next node in the linked list.
Implement a Linked List Class:
This class should have the following methods:
- add_task(task_name, duration, priority): Adds a new task to the end of the list.
- remove_task(task_name): Removes a task with the given name from the list.
- display_tasks(): Prints all tasks in the linked list in the order they appear.
- find_task(task_name): Searches for a task by name and returns its details.
- calculate_total_duration(): Calculates the total duration of all tasks in the current order.
- read_tasks_from_csv(file_path): Reads tasks from a CSV file and adds them to the linked list.
- reorder_tasks_by_priority(): Reorders the tasks in the linked list based on priority. (lowest priority number comes first).
- reorder_tasks_by_priority_duration(): Reorders the tasks in the linked list based on priority, then duration.
TIP: The reorder tasks are implemented by creating a new linked list to which the different nodes are added
based on priority. This means that you first need to implement two helper methods:
- sorted_insert_by_priority: This method has input a head (i.a linked list) and a node. The method adds the node to linked list by looking at the duration. Nodes with the lowest duration are added in the beginning of the LinkedList.
- sorted_insert_by_priority_duration: This method has input a head (i.a linked list) and a node. The method adds the node to linked list by looking first at the priority and next at duration. Nodes with the lowest priority and then duration are added in the beginning of the LinkedList.
Develop a Solution to Optimize Task Order
Given a series of tasks (see tasks.csv), reorder them to optimize for the shortest completion time. Consider both priority and duration.